I’m excited to announce the release of my fourth course at Pluralsight – “Integration Testing ASP.NET Core Applications: Best Practices“. This latest course focuses on using the ASP.NET Core integration testing framework.
Many developers will be familiar with the concepts of unit testing their applications. ASP.NET Core supports more in-depth end-to-end integration testing, with the help of a library which can be used in combination with xUnit test projects. The library provides a fixture which will run your application under test using an in-memory test server. This allows you to make requests to your application and to verify the responses and any expected side-effects.
Compared to unit tests, this allows much more of the application code to be tested together, which can rapidly validate the end-to-end behaviour of your service. These are also sometimes referred to as functional tests, since the definition of integration testing may be applied to more comprehensive multi-service testing as well. It’s entirely possible to test your applications in concert with their dependencies, such as databases or other APIs they expect to call.
In the course, I show how boundaries can be defined using fakes to test your application without external dependencies, which allows your tests to be run locally during development. Of course, you can avoid such fakes to test with some real dependencies as well. This form of in-memory testing can then easily be expanded to broader testing of multiple services as part of CI/CD workflows.
Producing these courses is a lot of work, but that effort is rewarded when people view the course and hopefully leave with new skills to apply in their work. If you have a subscription to Pluralsight already, I hope you’ll add this course to your bookmarks for future viewing. If you don’t yet have a subscription, you can sign up for access to this content and so many other fantastic courses.
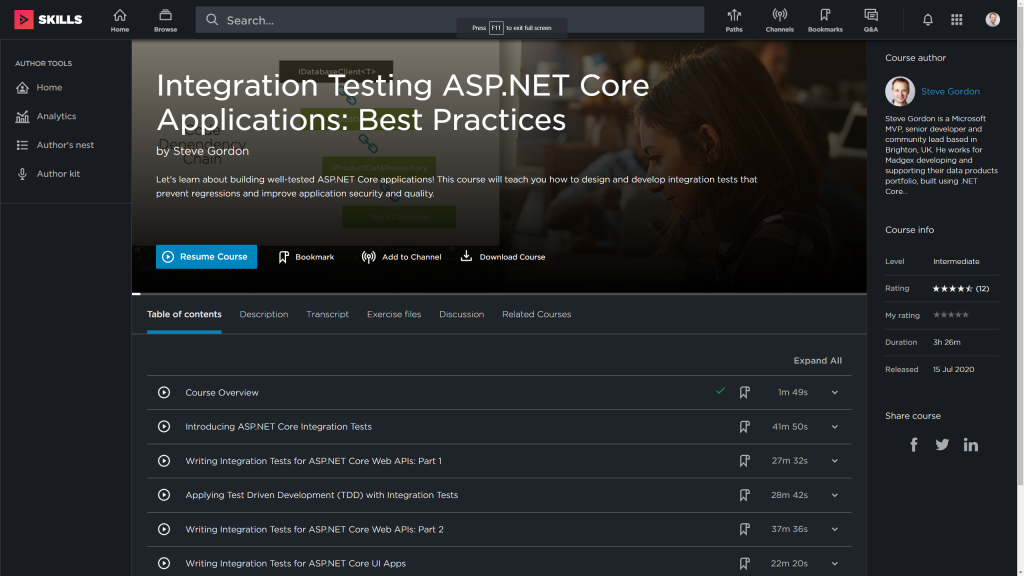
Module Summaries
Introducing ASP.NET Core Integration Tests
In this first module, I introduce the concept of integration tests. I then demonstrate how the ASP.NET Core testing library can be added to a project to begin the process of writing and running integration tests. These tests cause an ASP.NET Core Web API to be run in-memory, with requests being sent and the responses validated to confirm the expected behaviour.
We’ll cover:
- Introducing Integration Tests
- ASP.NET Core Integration Tests
- Creating an Integration Test Project
- Writing an Integration Test
- Running Integration Tests
- What Is the WebApplicationFactory?
Writing Integration Tests for ASP.NET Core Web APIs: Part 1
In this module, I expand on the basics and begin writing integration tests for an ASP.NET Core Web API project. I demonstrate how endpoints can be called using HTTP requests from the test methods. I focus on some of the challenges of asserting the correctness of JSON response content. I describe how the test client can be configured for different testing scenarios. This module concludes with examples of simplifying response assertions and how to validate for expected response headers.
We’ll cover:
- Testing Endpoints for GET Requests
- Improving Assertions for JSON Content
- Creating and Configuring the Test Client
- Simplifying Response Assertions
- Testing Response Headers
Applying Test Driven Development (TDD) with Integration Tests
In this module, I continue writing tests for an ASP.NET Core Web API but switch to a Test Driven Development paradigm. I define tests to describe new requirements for the API, implementing the feature to ensure those tests pass. I spend some time talking about the potential advantages of TDD before applying it in practical demos. I also talk about how we can avoid the need for external dependencies by using the test client to replace some services registered with the test host.
We’ll cover:
- Test Driven Development
- Applying Test Driven Development
- Defining Test Boundaries and Faking Dependencies
- Replacing Services in the Test Client
Writing Integration Tests for ASP.NET Core Web APIs: Part 2
In this module, I continue testing the Web API project. First, I show how a custom WebApplicationFactory can be used to apply a common test configuration to the test host. I discuss how to validate POST requests which include confirming that required input validation is applied and model binding works as expected. I introduce xUnit theory tests which can be used to test multiple inputs through the same test code. I dive into validating that requests trigger the expected side-effects, such as storing data to a database and focus on how to test middleware components and exceptions.
We’ll cover:
- Creating a Custom WebApplicationFactory
- Testing Model Binding and Input Validation for POST Requests
- Testing Multiple Conditions Using xUnit Theories
- Testing Responses for POST Requests
- Testing Success Responses
- Testing Side-Effects
- Testing Middleware
- Testing Exceptions
Writing Integration Tests for ASP.NET Core UI Apps
In this module, we move onto testing UI based applications, written using MVC or RazorPages approaches. I’ll compare some of the challenges and differences we must consider versus testing API projects. I introduce AngleSharp, an HTML parsing library we can use to help us validate the HTML content of requests. I conclude with a demonstration of testing dynamic page content which is controlled by the application configuration.
We’ll cover:
- Comparing APIs and UI Applications
- Touring the Integration Test Project
- Testing HTML Content with AngleSharp
- Testing Dynamic Page Content
Testing Advanced Requirements in ASP.NET Core UI Apps
In this final module, I cover more advanced testing of UI applications. I focus on some of the challenges around integration testing authentication and authorization in ASP.NET Core. I introduce the Entity Framework in-memory provider so that we can test code that stores data to a SQL database through Entity Framework Core. I spend some time refactoring some of the existing tests to make them easier to maintain and to re-use code between tests. I also show how to support testing of form POSTs where antiforgery tokens need to be dealt with.
We’ll cover:
- Configuring Redirects to Test Authentication
- Testing Authorization Requirements
- Configuring Entity Framework for In-memory Testing
- Testing Pages and Controllers Which Require Entity Framework
- Seeding and Resetting the Database within Tests
- Refactoring Test Code for Reusability
- Avoiding Brittle Tests
- Testing POST Requests by Handling Antiforgery Tokens
I hope that you find the content in this course useful and practical. Do let me know how you get on with the course. As I’ve applied in-memory integration tests to my projects, I’ve found that I need to write less individual unit tests and can be more confident that changes do not break my API contracts for consumers. When combined with other forms of testing, this helps to build robust software and avoid regressions in existing behaviour.
If you haven’t already, you can check out my previous courses:
- Dependency Injection in ASP.NET Core
- Using Configuration and Options in .NET Core and ASP.NET Core Apps
- Building ASP.NET Core Hosted Services and .NET Core Worker Services
Of course, you can also follow me directly on Pluralsight to get notified when I release new content.
Have you enjoyed this post and found it useful? If so, please consider supporting me: